updated 9 Sept 2014
This example finds the peak value of an analog sensor over time. It assumes the sensor is moving in a simple curve.
The peak value is initially set at zero. The program checks to see that the current value is greater than the current peak value. If so, then it saves the current value as a peak. If the current value is below a given threshold and the current peak value is above it, then the current peak is taken as the final peak. This example also includes a noise value, that allows you to adjust for sensor fluctuation.
The graph below gives you a visual sense of how the program operates. At readings 1, 2, and 3, the latest reading becomes the new peak. At reading 4, the peak remains unchanged because reading 4 is less than the last recorded peak. But the peak isn’t reported until reading 5, when the current reading goes below the threshold. This is the one disadvantage to this peak finding method: you don’t know you’ve had a peak until after it’s over.
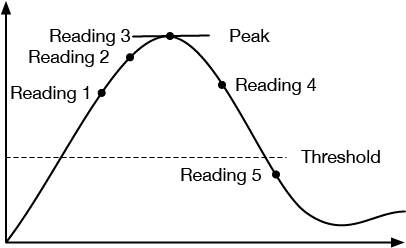
Continue reading “Peak Finding”